Open external links in new tab or window in Ghost
An easy way is to add a code snippet into our site using Ghost's code injection feature.
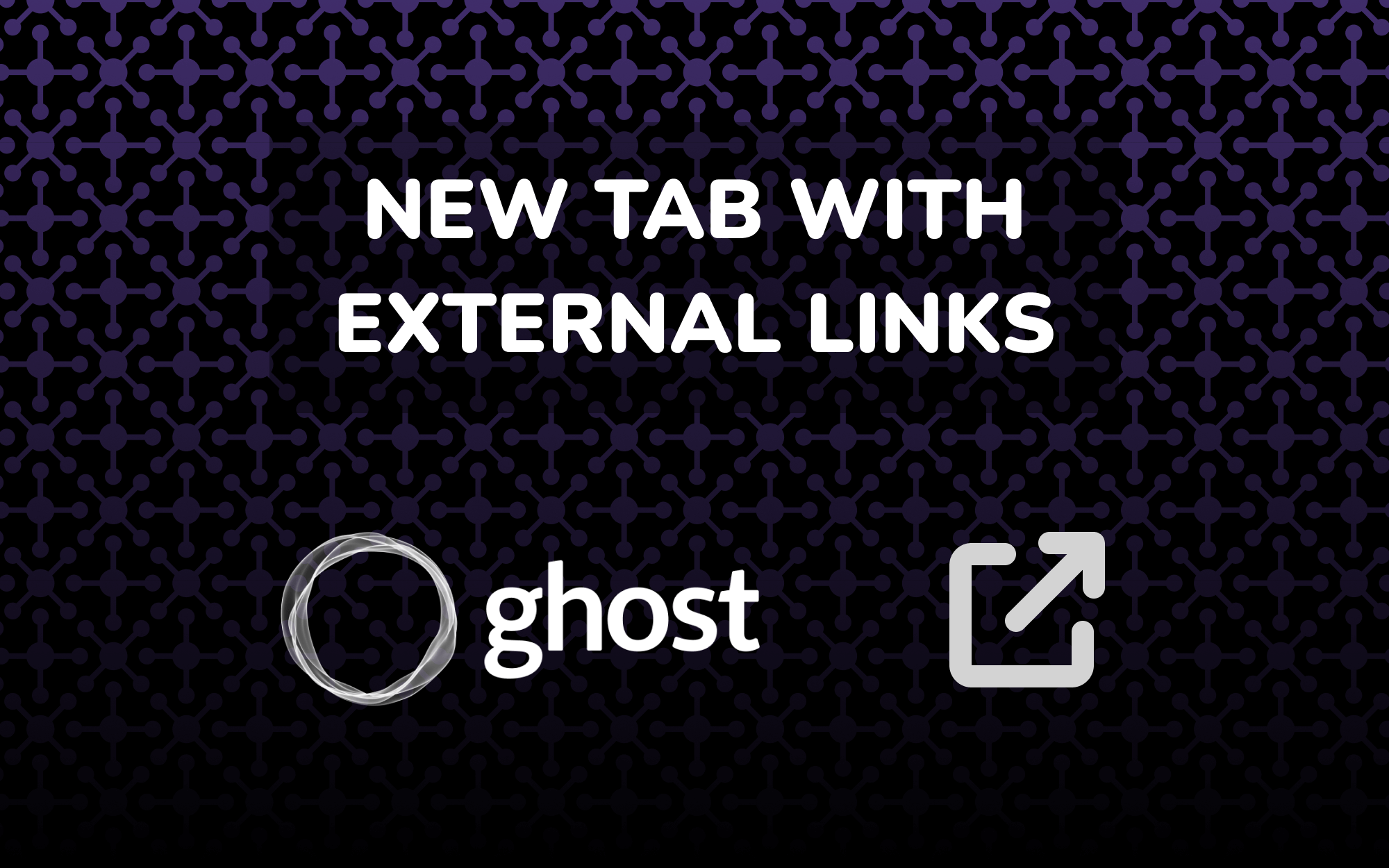
At the time of writing, the Ghost content editor does not support customizing of links, such as adding attributes to allow external links to open in a new tab or window. However, we can still achieve this through code injection (or editing theme files), so here's how.
What the code snippet does
First, we find all the link <a>
elements in the post content, and compare the url hostname value to that of the blog. Then, set the target
attribute to _blank
and rel
attribute to noreferrer noopener
(read this on why).
If you wish to include links in the menu navigation as well, check out the end of this article.
Option 1:
<script>
typeof $ !== 'undefined' && $(function () {
$('.gh-content a').filter(function () {
return this.hostname && this.hostname !== location.hostname;
}).attr({
target: '_blank',
rel: 'noreferrer noopener'
});
});
</script>
Option 2 (preferred):
- Does not require jQuery
- Backwards compatibility for browsers
<script>
(function () {
var list = document.querySelectorAll('.gh-content a');
for (var i = 0; i < list.length; i++) {
var a = list[i];
if ((a === null || a === void 0 ? void 0 : a.hostname) !== location.hostname) {
a.rel = "noopener noreferrer";
a.target = "_blank";
}
}
})();
</script>
This is a prudent approach so definitely my preferred choice.
document.querySelectorAll
here.Place the code snippet
- Go to your "Settings" page (the gear icon in the side bar)
- Under "Advanced", go to "Code injection"
- Copy and paste the code into under "Site Footer" (so that the code is executed after the DOM has loaded)
- Click "Save"
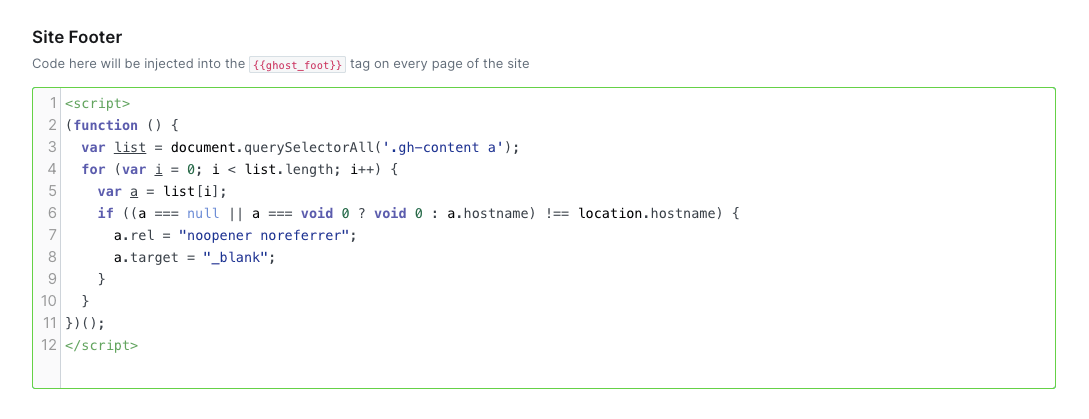
default.hbs
in the theme files if you have access to them. If you prefer to do so, add the code before {{ghost_foot}}
.What about links in navigation?
Add the .nav a
selector to the querySelectorAll
function like so, separated by a comma:
<script>
(function () {
var list = document.querySelectorAll('.gh-content a, .nav a');
for (var i = 0; i < list.length; i++) {
var a = list[i];
if ((a === null || a === void 0 ? void 0 : a.hostname) !== location.hostname) {
a.rel = "noopener noreferrer";
a.target = "_blank";
}
}
})();
</script>
Bonus: ES6 version
Array.from(document.querySelectorAll('.gh-content a'))
.filter(a => a?.hostname !== location.hostname).forEach(a => {
a.rel = "noopener noreferrer";
a.target = "_blank";
})