Exploring Javascript ES6 Features
Showcasing random ES6 stuff.
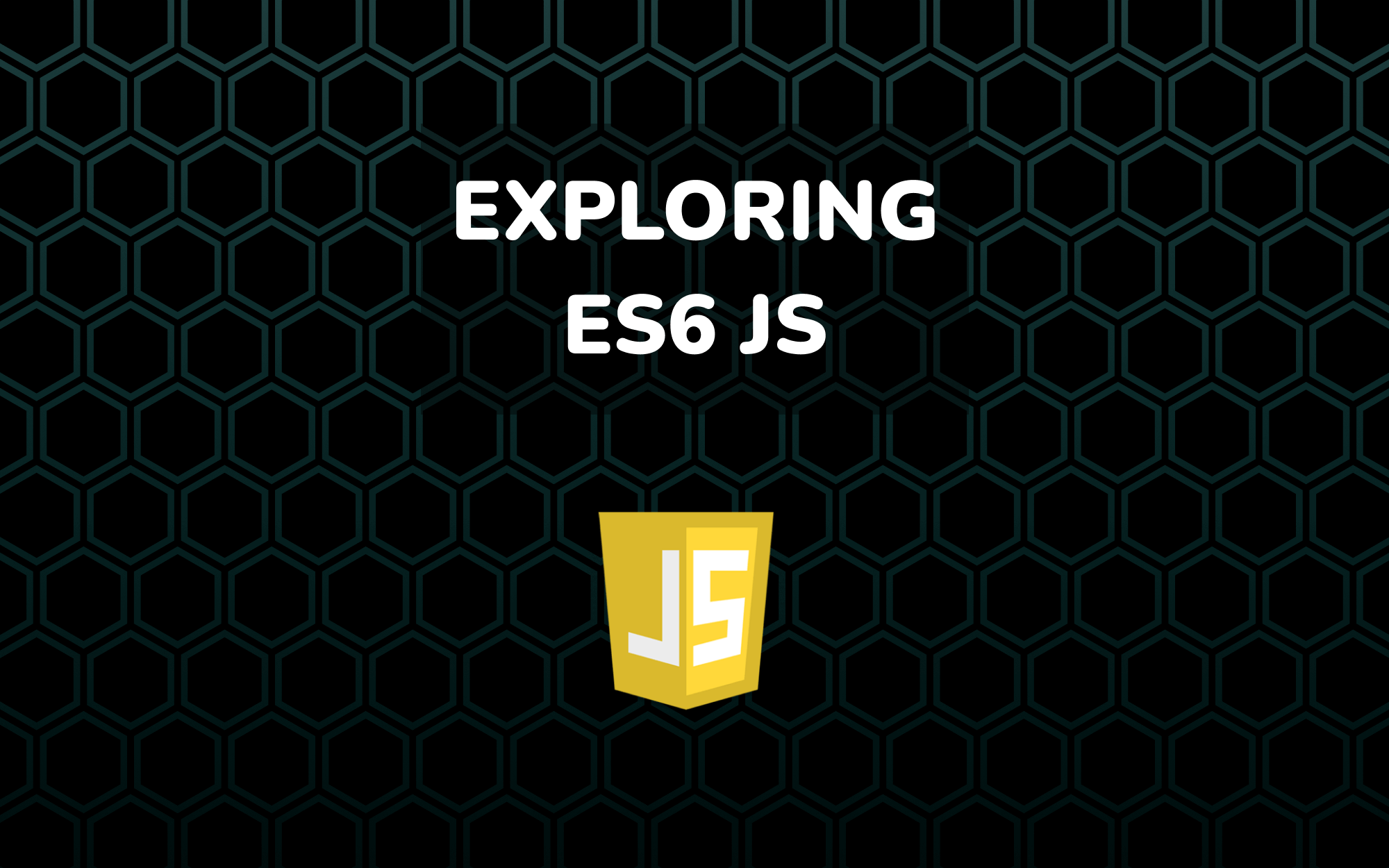
Just putting together a bunch of ES6 features.
Arrow function
const foo = x => x;
const foo = (x, y) => x + y;
const foo = (x, y) => ({x, y, z: x + y});
const foo = (x, y) => {
const z = [x, y];
// ...
return z;
}
// Currying
const add = (x) => (y) => (z) => x + y + z;
const addFiveTo = add(2)(3);
addFiveTo(3); // 8
Default value
const foo = num ?? 1;
const foo = (x = 1) => x;
const options = (userOptions) => ({...defaultOptions, ...userOptions});
const foo = ({ x = 1, y = 2 }) => x + y
const { x, y, z = 3 } = { x: 1, y: 2 };
const [ x, y, z = 3 ] = [1, 2]
Spread operator / Destructuring
const arr = [1, 2, 3];
arr = [...arr, 4];
arr = [4, ...arr];
arr = [...arr, ...arr2];
const [ first, ...others ] = arr;
const { x, ...others } = { x: 1, y: 2, z: 3 };
const foo = (x, y, z) => x + y + z, arr = [1, 2, 3];
foo(...arr);
const { x, y } = foo;
const foo = { x, y };
const z = {
x(){ foo(); },
y()( bar(); }
}
Rest operator
const foo = (...args) => args.join('');
Template literals
// Multi-line
const foo = `this is one line,
another line`;
// Interpolation
const foo = `the value is ${x}`;
// Tagged templates
const foo = (str, column, table) => db.select(x).from(table);
foo`select ${x} from ${table}`;